(Update March 6, 2014: The Junos “standard” proposal actually includes 3DES in both phase 1 and phase 2, still making it hard for VPNTracker to connect. What we need to do is create a custom proposal for each phase with only AES in it. I updated the text to reflect that.)
(Update November 11, 2014: VPN Tracker 7 failed for me if there were multiple remote networks configured. VPN Tracker 8 works fine with multiple remote networks, though, but you have to switch off the option under “advanced” that says “Establish a separate phase 2 tunnel for each remote network”. That option is new with VPN Tracker 8. I added that step in the text, but I didn’t update the screen shots. They’re all from VPN Tracker 7 still.)
So this is what I needed to accomplish: get one single SRX100 running Junos 12.1X44D20 to have a site-to-site VPN to another SRX100, while also having a dynamic VPN working with both Windows 7 clients and Mac OSX 10.9 clients. And I succeeded, except not for free. I’m not mentioning the site-to-site setup in what follows, since it does not interact with dynamic VPNs.
The SRX100 has a Windows VPN client built in, such that if you connect with a Win7 machine, it lets you log in using a web interface, then offers you a download of the Junos Pulse client, already completely configured for that SRX100. This works like a charm. The problem is that there is no Junos Pulse client for OSX, which I think is really weird. I love Juniper, but man, what’s up with this?
The client everyone recommends for this is VPN Tracker, a pretty expensive piece of very nice software from Equinux. But, of course, even though VPNTracker supports a truckload of firewalls, the SRX series is not among them. The software is very configurable, however, so even though there’s no guide, there’s a way.
First, take the lazy way and fire up the J-Web interface to the SRX100 (yes, I know, I lost all cred by doing this, but having to choose between self-respect and actually having a life, I finally crumbled, left the command line and took to the browser). To my defence, the J-Web interface is actually pretty darn good, at least compared to the Netscreen interface on the SSG5.
From experiments and a lot of searching on the interwebs, it’s clear there is a problem somehow with the DES and/or 3DES algorithms with the SRX100 and/or VPNTracker. They just can’t negotiate a phase 1 proposal, with VPNTracker failing in phase 1. The solution is to set the SRX100 to not use DES or 3DES, by selecting “Standard” in both “IKE Security Level” and “IPSec Security Level”.
The solution is to create a custom phase 1 proposal and phase 2 proposal that does not include DES or 3DES. In the SRX configuration, do:
set security ike proposal ike_prop_aesonly description "reduced proposal for vpntracker"
set security ike proposal ike_prop_aesonly authentication-method pre-shared-keys
set security ike proposal ike_prop_aesonly dh-group group2
set security ike proposal ike_prop_aesonly authentication-algorithm sha1
set security ike proposal ike_prop_aesonly encryption-algorithm aes-128-cbc
set security ike proposal ike_prop_aesonly lifetime-seconds 86400
set security ike policy ike_pol_wizard_dyn_vpn proposals ike_prop_aesonly
Don’t forget to remove the policy-set standard you had from before. The “ike_pol_wizard_dyn_vpn” can have another name on your system, of course.
and…
set security ipsec proposal ipsec_prop_aesonly description "reduced proposal for vpntracker"
set security ipsec proposal ipsec_prop_aesonly protocol esp
set security ipsec proposal ipsec_prop_aesonly authentication-algorithm hmac-sha1-96
set security ipsec proposal ipsec_prop_aesonly encryption-algorithm aes-128-cbc
set security ipsec proposal ipsec_prop_aesonly lifetime-seconds 3600
set security ipsec policy ipsec_pol_wizard_dyn_vpn proposals ipsec_prop_aesonly
Also, choose group 2 in IPSec Perfect Forward Secrecy (I haven’t experimented with other values here). With these choices, there’s no use of DES or 3DES. Note the IKE Preshared key and Remote Identity values. In the next screen, you choose users and stuff, and I’m not going to show you mine. Nothing there has a bearing on this description anyway.
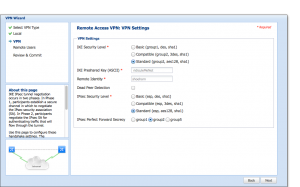
So, with this set, first try to log in using Win7, download the Junos Pulse client and connect. This should work without anything else but your login credentials that you set in the screen “Remote Users” (that I didn’t show) in the VPN Wizard on the SRX100.
Now, back to VPNTracker. In the “Basic screen” of your connection setup, you enter the following:
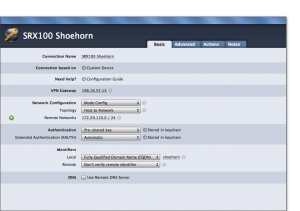
And in the second, “Advanced”, you enter the following:
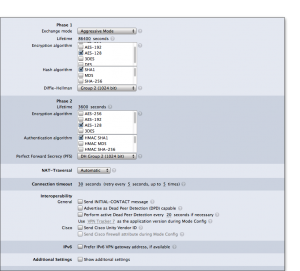
Notable items in the first of the two screens (“Basic”) are:
- Network configuration: “Mode Config”
- Identifiers, local: “FQDN”, with the “shoehorn” name you entered in the SRX100 VPN Wizard as “Remote Identity”
- Identifiers, remote: “Don’t verify”
Notable items in the second (“Advanced”) screen, where we take care to only select the algorithms included in the Junos SRX100 “Standard” set, which is AES-128, SHA-1, and DH Group2 in both phase 1 and phase 2, and DH Group2 for PFS in phase 2:
- Exchange mode: Aggressive
- Phase 1 encryption: AES-128 only
- Phase 1 hash: SHA1 only
- Diffie-Hellman: group 2
- Phase 2 encryption: AES-128 only
- In VPN Tracker 8, there is here a checkbox “Establish a separate phase 2 tunnel for each remote network”. This should be off.
- Authentication: HMAC SHA1 only
- PFS: DH Group 2
There’s one last, hard to find, little detail: you have to change the ike-user-type on the SRX100 from “shared-ike-id”, as the wizard generated it, to “group-ike-id”, through the commandline on the SRX100, assuming your SRX100 VPN Wizard generated the gateway name to be “gw_wizard_dyn_vpn”, which it probably did, like so:
set security ike gateway gw_wizard_dyn_vpn dynamic ike-user-type group-ike-id
commit
Interestingly, you don’t have to change your remote identifier in any way, neither in the SRX100 or the VPNTracker. From now on, you can connect.
Now, amazingly, you can connect with VPNTracker from OSX 10.9 while at the same time using the Junos Pulse client from Win7. All you have to do now is cough up $100 or $200 for VPNTracker (depending on version).
The SRX100 comes with a default two simultaneous dynamic VPN connections. You can get five connections with an extra license (SRX-RAC-5-LTU) for around $150, but I don’t know if that leaves you with a total of five or seven simultaneous connections.